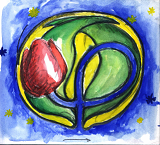 |
Tulip
5.6.0
Large graphs analysis and drawing
|
20 #ifndef _PERSPECTIVE_H
21 #define _PERSPECTIVE_H
23 #include <tulip/PluginContext.h>
24 #include <tulip/Plugin.h>
25 #include <tulip/TlpQtTools.h>
28 #include <QVariantMap>
40 static const std::string PERSPECTIVE_CATEGORY =
"Perspective";
51 QMainWindow *mainWindow;
52 TulipProject *project;
54 QVariantMap parameters;
86 QSet<QString> _reservedProperties;
87 QTcpSocket *_agentSocket;
88 unsigned int _perspectiveId;
90 void sendAgentMessage(
const QString &);
91 void notifyProjectLocation(
const QString &path);
135 bool checkSocketConnected();
138 enum ProgressOption {
139 NoProgressOption = 0x0,
144 Q_DECLARE_FLAGS(ProgressOptions, ProgressOption)
146 std::
string category()
const override {
147 return PERSPECTIVE_CATEGORY;
149 std::string
icon()
const override {
150 return ":/tulip/gui/icons/32/plugin_controller.png";
173 template <
typename T>
175 return dynamic_cast<T *
>(instance());
201 progress(ProgressOptions options = ProgressOptions(IsPreviewable | IsStoppable | IsCancellable));
206 virtual void usage(std::string &usage_str)
const {
207 usage_str =
"No options for this perspective.";
213 QMainWindow *mainWindow()
const;
221 bool isReservedPropertyName(QString name);
226 void registerReservedProperty(QString);
232 virtual void redrawPanels(
bool center =
false) = 0;
238 virtual void centerPanelsForGraph(
tlp::Graph *);
241 emit resetWindowTitle();
249 return _restartNeeded;
256 instance()->displayStatusMessage(msg);
270 static void redirectStatusTipOfMenu(QMenu *menu);
277 const QString &msg) {
278 instance()->logMessage(type, context, msg);
285 instance()->displayLogMessages();
292 static void setStyleSheet(QWidget *w);
298 static QString styleSheet();
311 void resetWindowTitle();
317 void showPluginsCenter();
323 void showFullScreen(
bool f);
328 void showProjectsPage();
333 void showAboutPage();
340 void showTrayMessage(
const QString &s);
348 void showErrorMessage(
const QString &title,
const QString &s);
354 virtual void openProjectFile(
const QString &path);
360 void createPerspective(
const QString &name);
366 void showStatusTipOf(QAction *action);
371 virtual void displayStatusMessage(
const QString &s);
376 virtual void clearStatusMessage();
386 virtual void logMessage(QtMsgType,
const QMessageLogContext &,
const QString &) {}
388 Q_DECLARE_OPERATORS_FOR_FLAGS(Perspective::ProgressOptions)
391 #endif //_PERSPECTIVE_H
QMainWindow * _mainWindow
The main window on which the perspective should build the GUI.
virtual bool terminated()
Called when the user wants to close the application.
virtual void usage(std::string &usage_str) const
usage Displays a usage message when called from the tulip_perspective executable
static void showLogMessage(QtMsgType type, const QMessageLogContext &context, const QString &msg)
a static function to log a message see qInstallMessageHandler
virtual void displayLogMessages()
a virtual function to display the whole logs
Contains runtime parameters for a plugin.
static void showLogMessages()
a static function to display the log messages
TulipProject * _project
The project associated to this perspective. This project can be empty or contain data depending on ho...
Top-level interface for plug-ins.
A Perspective is a Tulip plugin that completely re-defines the user interface.
static void showStatusMessage(const QString &msg)
a static function to ease the display of status messages
std::string icon() const override
The icon (preferably a thumbnail) of the plugin.
A context data structure for tlp::Perspective instances.
QVariantMap _parameters
Contains extra parameters that have not been parsed by the overleying system. Those are considered to...
virtual void logMessage(QtMsgType, const QMessageLogContext &, const QString &)
a virtual function to log a message
bool needRestart()
a function to indicate restart
QString tlpStringToQString(const std::string &toConvert)
Convert a Tulip UTF-8 encoded std::string to a QString.
static void showStatusMessage(const std::string &msg)
a static function to ease the display of status messages
static T * typedInstance()
PluginProcess subclasses are meant to notify about the progress state of some process (typically a pl...