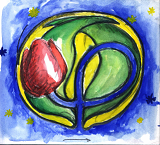 |
Tulip
5.6.0
Large graphs analysis and drawing
|
25 #include <tulip/tulipconf.h>
26 #include <tulip/Iterator.h>
27 #include <tulip/Node.h>
58 enum EventType { TLP_DELETE = 0, TLP_MODIFICATION, TLP_INFORMATION, TLP_INVALID };
62 EventType type()
const {
150 static void holdObservers();
162 static void unholdObservers();
171 return _oHoldCounter;
204 void addObserver(
Observable *
const observer)
const;
213 addObserver(&observer);
223 void addListener(
Observable *
const listener)
const;
233 addListener(&listener);
242 void removeObserver(
Observable *
const observer)
const;
251 removeObserver(&observer);
260 void removeListener(
Observable *
const listener)
const;
269 removeListener(&listener);
276 unsigned int getSent()
const;
282 unsigned int getReceived()
const;
288 unsigned int countObservers()
const;
294 unsigned int countListeners()
const;
321 static unsigned int getScheduled(
tlp::node n);
336 static const tlp::VectorGraph &getObservableGraph();
360 void sendEvent(
const Event &message);
371 virtual void treatEvents(
const std::vector<Event> &events);
380 virtual void treatEvent(
const Event &message);
398 void observableDeleted();
412 bool hasOnlookers()
const;
415 enum OBSERVABLEEDGETYPE { OBSERVABLE = 0x01, OBSERVER = 0x02, LISTENER = 0x04 };
425 mutable bool queuedEvent;
440 unsigned int received;
475 void addOnlooker(
const Observable &obs, OBSERVABLEEDGETYPE type)
const;
491 void removeOnlooker(
const Observable &obs, OBSERVABLEEDGETYPE type)
const;
503 static unsigned int _oHoldCounter;
508 static bool _oDisabled;
514 static void updateObserverGraph();
522 bool isBound()
const {
void removeListener(Observable &listener) const
Removes a listener from this object.
void addListener(Observable &listener) const
Adds a Listener to this object.
static void disableEventNotification()
disable the whole event notification mechanism Until a call to enableEventNotification(),...
PropertyInterface describes the interface of a graph property.
bool isValid() const
isValid checks if the node is valid. An invalid node is a node whose id is UINT_MAX.
Event is the base class for all events used in the Observation mechanism.
static unsigned int observersHoldCounter()
observersHoldCounter gives the number of times holdObservers() has been called without a matching unh...
static void holdObservers()
Holds back all events until Observable::unholdObservers() is called.
The ObserverHolder class is a convenience class to automatically hold and unhold observers....
Interface for Tulip iterators. Allows basic iteration operations only.
static void unholdObservers()
Sends all held events to the Observers.
The Observable class is the base of Tulip's observation system.
void removeObserver(Observable &observer) const
Removes an observer from this object.
The node struct represents a node in a Graph object.
static void enableEventNotification()
re-enable the whole event notification mechanism All events sent since a previous call to enableEvent...
void addObserver(Observable &observer) const
Adds an Observer to this object.